This is one of those code warrior snippets with two-lines that one never seems to find around the Internet, so I'm posting here for reference.
If you have a String and want to remove all the characters inside the string that are not numbers, this works:
public static int justNumbers(String input){
String temp = input.replaceAll("[^0-9]", "");
return Integer.parseInt(temp);
}
Seems pretty simple doesn't it? Now look around the Internet and see the other techniques being proposed.
Hope this helps you.
TripleCheck, Beanshell and SPDX
I've been using most of my free time to develop the new SPDX tool. It started as command line tool and then moved up to a desktop edition.
Not happy with the usual static forms that you see on normal GUI applications, I decided to add a flavor of HTML inside the Swing containers. The result was great. Then, I wanted to make sure that other people could change the code. It is no fun when you always need to compile things around when all you want is to just change a few settings.
Beanshell was used for scripting the plugins. Editing Beanshell scripts is not so much fun when programming errors happen. There is no syntax checking, no assistance to write code in the same smart manner as you see in modern IDEs available today.
The answer was creating what I call of Java-like beanshell. Moved things up to a state when you can comfortably write a Beanshell script exactly in the same fashion as it was a normal Java file. This was one heck of a productivity boost. Suddenly, writing dynamic scripts was much easier thanks to this neat trick.
Then came the next step, what about the web? I had been avoiding the idea of writing a web application. Basically because it places some restrictions such as requiring users to open a network port on their machines, which might not be possible on the case of many people working on machines at their companies.
Solution? Do a bridge between desktop and browser world. Today I completed this goal. The screenshot below demonstrates how it is possible to write java-like beanshell scripts that will behave exactly the same, regardless of the user access the program from the desktop or web edition.
It is quite a mix. The best of the Java infrastructure (OS agnostic, robust language syntax, huge repository of available libraries and connectivity), the best of Beanshell (scripting just like PHP) and the best of two user interfaces: Desktop and Web Browsers.
Inside the beanshell script, a method is declared with the object that contains all the details about the request. Writing a plugin for the log viewer was a simple as this:
/**
* Displays a basic log of what has been happening
* @param request The request from the end-user
*/
public void showPage(WebRequest request){
// get all the messages since the beginning
String result = log.getMessagesSince(0);
// replace the break lines with an HTML break line
result = result.replace("\n", html.br);
// write everything for the user to read
request.setAnswer(result);
}
Really simple, really fun.
I'm doing this code for the TripleCheck software that generates SPDX documents. If there is interest, I can later release this particular part of the code as project of its own for those who are interested in:
- Writing apps that work both on a Swing or Web browser
- Writing apps based on a Java-like scripting language that can be written from a normal IDE
- Keeping things simple and small (the whole software is less than 10Mb)
:-)
BeanShell unleashed
I'm a big fan of BeanShell.
Not only I enjoy the fact that this was the first scripting language available for the Java platform, as I really enjoy the phenomenal possibility of allowing the users of my software to make changes.
Phenomenal is indeed a suited word. The potential of BeanShell is unleashed on the fact that after a given software is compiled it gets very complicated for end-users to customize a tool. So, this nifty scripting language does what is needed.
There is however one bogging weakness. There is no proper IDE available for writing code in BeanShell, let alone an IDE that allows integrating the scripting language directly onto the libraries from our software. You get code that anyone can modify with a simple text editor and at the same time abdicate the comfort of modern day development environments since not so many folks play around with this kind of scripted language.
I've been dwelling with this IDE problem for more than two years now across different products being developed. Tried different approaches but the result was still lacking in terms of quality, until today. :-)
For a while now that I imagined how it would be possible to combine an IDE (say NetBeans) with BeanShell. The basic Java language is equal to some degree on both sides, should be noted that BeanShell is no longer a developed product and the language stopped on Java 5. Still, what would happen if we renamed the common beanshell extension (.bsh) to a .java extension?
And so I did. It was easy to configure NetBeans to accept an additional folder as source. The problem are the small differences between the two worlds. What I did was quite simple, I created a new class and then dropped the code from a BeanShell script inside.
Eventually, I noticed that it was possible to parse and change the lines of the script file in RAM, modifying the Java code to be acceptable as BeanShell. Not many changes were needed:
- Removing the declaration of "package"
- Deleting "@override" sentences
- Modifying the "class" sentence to create an object of the Plugin class
After these minor changes that are automated when running a script, the Java code becomes BeanShell code. Awesome! I can keep using the same IDE while at the same writing scripts with a syntax checker and all the fancy tools that allow adding up so much code automatically nowadays.
I'm not planning in doing a separate product to demonstrate this kind of functionality in action, but if you're interested then do let me know and I will do a small prototype.
BeanShell rocks! :-)
Not only I enjoy the fact that this was the first scripting language available for the Java platform, as I really enjoy the phenomenal possibility of allowing the users of my software to make changes.
Phenomenal is indeed a suited word. The potential of BeanShell is unleashed on the fact that after a given software is compiled it gets very complicated for end-users to customize a tool. So, this nifty scripting language does what is needed.
There is however one bogging weakness. There is no proper IDE available for writing code in BeanShell, let alone an IDE that allows integrating the scripting language directly onto the libraries from our software. You get code that anyone can modify with a simple text editor and at the same time abdicate the comfort of modern day development environments since not so many folks play around with this kind of scripted language.
I've been dwelling with this IDE problem for more than two years now across different products being developed. Tried different approaches but the result was still lacking in terms of quality, until today. :-)
For a while now that I imagined how it would be possible to combine an IDE (say NetBeans) with BeanShell. The basic Java language is equal to some degree on both sides, should be noted that BeanShell is no longer a developed product and the language stopped on Java 5. Still, what would happen if we renamed the common beanshell extension (.bsh) to a .java extension?
And so I did. It was easy to configure NetBeans to accept an additional folder as source. The problem are the small differences between the two worlds. What I did was quite simple, I created a new class and then dropped the code from a BeanShell script inside.
Eventually, I noticed that it was possible to parse and change the lines of the script file in RAM, modifying the Java code to be acceptable as BeanShell. Not many changes were needed:
- Removing the declaration of "package"
- Deleting "@override" sentences
- Modifying the "class" sentence to create an object of the Plugin class
After these minor changes that are automated when running a script, the Java code becomes BeanShell code. Awesome! I can keep using the same IDE while at the same writing scripts with a syntax checker and all the fancy tools that allow adding up so much code automatically nowadays.
I'm not planning in doing a separate product to demonstrate this kind of functionality in action, but if you're interested then do let me know and I will do a small prototype.
BeanShell rocks! :-)
New laptop - Lenovo U310 (i7 version)
After fighting many battles with the help of my faithful Toshiba R630 over the past years, I've now placed it to rest and got a new machine.
Before getting into details of the new machine, I have to say that Toshiba has really lived up to my expectations. It has been exactly three years using the laptop nearly on daily basis for most of my day-time work:
So, why have I got a new machine then?
As part of my work I need to run some very specific tools. One of them has a database of over 450Gb and requires (at minimum) some 8Gb of RAM to be able of functioning. It is a software intended for server machines. However, I wanted to have something portable and independent of network connectivity. Initially I just bought an 8Gb memory card and a new SSD upgrade for my old laptop but unfortunately this was still not enough to make the software perform as necessary, so a new machine seemed like a reasonable approach.
I wanted to keep costs at minimum, I wanted an i7 CPU processor, some 8Gb of RAM and I wanted the new machine needed to look "nice". Please don't burn me for wanting laptops to look nice, just remember that I will work with the same machine on daily basis for several years in a row. When possible to decide which machine to use then I will give some selection points to aesthetics.. :-)
After consideration of the machines available right now, I've found the option from Lenovo as the most interesting one for my case.
The specs were nice:
I couldn't find many reviews online about this specific model. It seems that all the reviews that I could find discussed only the i5 CPU with 4Gb of RAM from an older model. So, it was kind of a "leap of faith" to trust fully on the specifications from the manufacturer. In either case, not much risk was involved since I am allowed to return the equipment in case it doesn't perform to what was needed.
So, I've got this machine and my first reaction when unpacking was "wow, it is small". Over these years I should already got used to how fast technology packs such a hefty power into smaller and smaller devices but quite honestly this is something that I enjoy getting surprised about.
The initial boot went ok, Windows worked. This is my first machine with Windows 8 and I've got to say that I find the lack of a start menu disturbing. I've read that with Windows 8.1 there is some kind of alternative being added, let's see. My goal wasn't having a Windows 8 machine, what I required was a Linux operating system to be up and running as fast as possible to test the software.
My weapon of choice was Linux Mint. Optical drive is not available on this laptop but typically you can install Ubuntu/Mint straight from Windows. I did a mistake here. On my desk table was already available an ISOstick with Mint available as ISO and I decided to install it from there to save some time. The big problem is that later during the reboot it launched the CD installer and from there I decided (on my own will) to ignore the Windows installer, causing later to have two entries on the boot menu. Lesson learned, next time I will just use the ISOstick with Mint to permit running Linux in full speed.
On newer laptops, I should note that it might help to try disabling the "Fast boot" and "secure boot" services from the BIOS setup in case you're not getting the boot up to happen.
With an SSD equipped on this laptop, I decided to use this disk as default location for the Mint install. Also my first time running a Linux install under SSD. Under an hour I had my basic Linux setup running amazingly fast from SSD on a machine with an i7 core and 8Gb RAM. All basic drivers worked out of the box (WIFI, sound, screen and even shortcut keys). Didn't tested the web camera or other details, might also be working. My only complaint is that the mouse pad is very sensitive and quite often the mouse tracker moves (and changes the text cursor position) while writing text because of your wrists touching it. The Windows driver is ignoring this kind of design quirk but the Ubuntu driver was not handling this quirk by default (will later try to find how to get this point addressed).
From there, got the older versions of MySQL 5.1 and the Oracle JDK 1.6 working. Copied over the database and then the software functioned as intended. I was happy, problem solved.
I like this machine. It is more silent than my older Toshiba laptop and still strong enough to act like an enterprise machine when needed. I didn't had much contact with Lenovo before, only knew them after the acquisition of the ThinkPad product line from IBM and was quite impressed to see such a nice ensemble available on this equipment. Let's see if it will last long on my hands. :-)
Testing Windows 8
After a while, I went back to the Windows 8 install to see what I was missing with the new OS. There are some things I like about the metro design. It is great to use touch for displaying pictures and talking about them with others. I found the Windows market place quite poor in comparison to Android's. The worst thing for me was not knowing how to "search" for apps. Had to look around the web to discover that I needed to press "Windows-key + Q" to call the search box or use swipe the finger to bring about the right-side menu with the search bar. Lesson learned, I just wish there was some kind of visual hint or button for this action.
The lack of a start menu was indeed a bother. Took me quite a while to rebuild some of my often used shortcuts for Windows utils. What I hated most is the fact that I can't quickly type from the start menu to find a program like before. I've ended finding "Start 8" from IOBit as alternative, this software impressed me because it addresses exactly what I wanted. The problem: it seems to be powered by infamous "push ads" and has already asked me to install some "system cleaners" that I would gladly live without. I'm still keeping it installed, hope it doesn't get worse or another option needs to be found.
At the end of the day the biggest surprise was something unexpected. I was in bed with my better half, she wanted to see something nice on the PC before falling asleep. Out of her own initiative she clicked on the "bing travels" icon to see some holiday pictures. Inside this metro app came the option of looking at some 360 degree pictures from famous locations. I was impressed. The touch screen was so responsive and you could use the fingers just like in "Minority report" to explore the given detail of a location. What an unexpected surprise, the future is arriving at quick pace indeed. In the end, we both enjoyed doing some tourism without leaving the house. Definitively a plus point there for the nice surprise.
The aftermath?
New machine, plenty of work. Let's move. :-)
Before getting into details of the new machine, I have to say that Toshiba has really lived up to my expectations. It has been exactly three years using the laptop nearly on daily basis for most of my day-time work:
- Battery holds a full charge and I can work some four hours without an electricity plug
- Original Windows installation that came with the computer is still working great. Updates and normal usage over these years haven't made me need to remove it away
- DVD drive got broken, no longer works
- From the outside, still looks as a modern machine
So, why have I got a new machine then?
As part of my work I need to run some very specific tools. One of them has a database of over 450Gb and requires (at minimum) some 8Gb of RAM to be able of functioning. It is a software intended for server machines. However, I wanted to have something portable and independent of network connectivity. Initially I just bought an 8Gb memory card and a new SSD upgrade for my old laptop but unfortunately this was still not enough to make the software perform as necessary, so a new machine seemed like a reasonable approach.
I wanted to keep costs at minimum, I wanted an i7 CPU processor, some 8Gb of RAM and I wanted the new machine needed to look "nice". Please don't burn me for wanting laptops to look nice, just remember that I will work with the same machine on daily basis for several years in a row. When possible to decide which machine to use then I will give some selection points to aesthetics.. :-)
After consideration of the machines available right now, I've found the option from Lenovo as the most interesting one for my case.
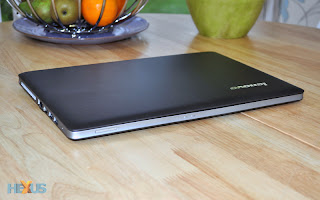
- i7 CPU
- 8Gb RAM
- 500GB HDD
- 26Gb SSD
- Touch screen
- Windows 8
- Compact machine (not a bulky laptop)
- Stylish look (dark-blue metal)
- Under 900 Euros
I couldn't find many reviews online about this specific model. It seems that all the reviews that I could find discussed only the i5 CPU with 4Gb of RAM from an older model. So, it was kind of a "leap of faith" to trust fully on the specifications from the manufacturer. In either case, not much risk was involved since I am allowed to return the equipment in case it doesn't perform to what was needed.
So, I've got this machine and my first reaction when unpacking was "wow, it is small". Over these years I should already got used to how fast technology packs such a hefty power into smaller and smaller devices but quite honestly this is something that I enjoy getting surprised about.
The initial boot went ok, Windows worked. This is my first machine with Windows 8 and I've got to say that I find the lack of a start menu disturbing. I've read that with Windows 8.1 there is some kind of alternative being added, let's see. My goal wasn't having a Windows 8 machine, what I required was a Linux operating system to be up and running as fast as possible to test the software.
My weapon of choice was Linux Mint. Optical drive is not available on this laptop but typically you can install Ubuntu/Mint straight from Windows. I did a mistake here. On my desk table was already available an ISOstick with Mint available as ISO and I decided to install it from there to save some time. The big problem is that later during the reboot it launched the CD installer and from there I decided (on my own will) to ignore the Windows installer, causing later to have two entries on the boot menu. Lesson learned, next time I will just use the ISOstick with Mint to permit running Linux in full speed.
On newer laptops, I should note that it might help to try disabling the "Fast boot" and "secure boot" services from the BIOS setup in case you're not getting the boot up to happen.
With an SSD equipped on this laptop, I decided to use this disk as default location for the Mint install. Also my first time running a Linux install under SSD. Under an hour I had my basic Linux setup running amazingly fast from SSD on a machine with an i7 core and 8Gb RAM. All basic drivers worked out of the box (WIFI, sound, screen and even shortcut keys). Didn't tested the web camera or other details, might also be working. My only complaint is that the mouse pad is very sensitive and quite often the mouse tracker moves (and changes the text cursor position) while writing text because of your wrists touching it. The Windows driver is ignoring this kind of design quirk but the Ubuntu driver was not handling this quirk by default (will later try to find how to get this point addressed).
From there, got the older versions of MySQL 5.1 and the Oracle JDK 1.6 working. Copied over the database and then the software functioned as intended. I was happy, problem solved.
I like this machine. It is more silent than my older Toshiba laptop and still strong enough to act like an enterprise machine when needed. I didn't had much contact with Lenovo before, only knew them after the acquisition of the ThinkPad product line from IBM and was quite impressed to see such a nice ensemble available on this equipment. Let's see if it will last long on my hands. :-)
Testing Windows 8
After a while, I went back to the Windows 8 install to see what I was missing with the new OS. There are some things I like about the metro design. It is great to use touch for displaying pictures and talking about them with others. I found the Windows market place quite poor in comparison to Android's. The worst thing for me was not knowing how to "search" for apps. Had to look around the web to discover that I needed to press "Windows-key + Q" to call the search box or use swipe the finger to bring about the right-side menu with the search bar. Lesson learned, I just wish there was some kind of visual hint or button for this action.
The lack of a start menu was indeed a bother. Took me quite a while to rebuild some of my often used shortcuts for Windows utils. What I hated most is the fact that I can't quickly type from the start menu to find a program like before. I've ended finding "Start 8" from IOBit as alternative, this software impressed me because it addresses exactly what I wanted. The problem: it seems to be powered by infamous "push ads" and has already asked me to install some "system cleaners" that I would gladly live without. I'm still keeping it installed, hope it doesn't get worse or another option needs to be found.
At the end of the day the biggest surprise was something unexpected. I was in bed with my better half, she wanted to see something nice on the PC before falling asleep. Out of her own initiative she clicked on the "bing travels" icon to see some holiday pictures. Inside this metro app came the option of looking at some 360 degree pictures from famous locations. I was impressed. The touch screen was so responsive and you could use the fingers just like in "Minority report" to explore the given detail of a location. What an unexpected surprise, the future is arriving at quick pace indeed. In the end, we both enjoyed doing some tourism without leaving the house. Definitively a plus point there for the nice surprise.
The aftermath?
New machine, plenty of work. Let's move. :-)
Ubuntu and Richard Stallman
Typically, I enjoy participating around the web in forum sites, mailing lists and just any other normal social activities.
It is a good experience for the most part, can say that I've learned so much from plain interaction with other folks around the web. However, the current situation with Ubuntu turned into a distribution that automatically logs your activity and sells this information to other parties is something that deeply upsets me, mostly because I really hoped that the Ubuntu project would not turn evil.
This just gets worse with Unity. A user interface that goes against any comfort for the end user and that on the most recent editions of Ubuntu reveals the reasons why it was so oddly designed: mixing advertisements with the tools that people are looking for.
Very sad, very disappointing. The border line of this situation was looking on the announcement of an article at ZDnet entitled "Ubuntu 13.10 Review: A great Linux desktop gets better".
Using the term "review" and "better" as head line for the article is a shame for ZDnet, should be better called "marketing" with the author minimizing the critics to the Unity interface or selling of data to Amazon while going as far as praising the install procedure to bring users into the Ubuntu One cloud storage with "5GBs of no-cost storage. The commercial version, at $39.95, gives you 20GBs" .
Seriously. On a time when governments around the world are exposed for obvious enticing of people to place more of their data in the "clouds" where everyone can bypass the basic privacy rights of end-users, this kind of thing really brings me to disappointment.
Even worse to see normal people making the work easier for these marketeers and to support them completely. I was frustrated. Like a religious person in Brazil (Padre António Vieira) once said back in the 16th century when criticizing the slavery practice: "If nobody cares about what I preach, I will preach to the fish but will not stay silent". Reminded me of what Richard Stallman once wrote about Ubuntu some time ago and how it still made sense today. I understood better the motivation of Stallman and Vieira to write such words, it is disappointment.
So, feeling overwhelmed with people replying on the forum topic where Unity is great, where the loss of privacy is not true despite recent leaks. I felt quite alone on this question and wrote a message to Richard Stallman. To my surprise, he replied with calming words of advice.
So. Lesson learned. I stopped arguing with those folks on the forum topic after feeling that there was nothing more of productive to be added from my participation. I will nevertheless preach to the fishes with the hope that some day in the future we can have Ubuntu as a "good-guy" again.
It is a good experience for the most part, can say that I've learned so much from plain interaction with other folks around the web. However, the current situation with Ubuntu turned into a distribution that automatically logs your activity and sells this information to other parties is something that deeply upsets me, mostly because I really hoped that the Ubuntu project would not turn evil.
This just gets worse with Unity. A user interface that goes against any comfort for the end user and that on the most recent editions of Ubuntu reveals the reasons why it was so oddly designed: mixing advertisements with the tools that people are looking for.
Very sad, very disappointing. The border line of this situation was looking on the announcement of an article at ZDnet entitled "Ubuntu 13.10 Review: A great Linux desktop gets better".
Using the term "review" and "better" as head line for the article is a shame for ZDnet, should be better called "marketing" with the author minimizing the critics to the Unity interface or selling of data to Amazon while going as far as praising the install procedure to bring users into the Ubuntu One cloud storage with "5GBs of no-cost storage. The commercial version, at $39.95, gives you 20GBs" .
Seriously. On a time when governments around the world are exposed for obvious enticing of people to place more of their data in the "clouds" where everyone can bypass the basic privacy rights of end-users, this kind of thing really brings me to disappointment.
Even worse to see normal people making the work easier for these marketeers and to support them completely. I was frustrated. Like a religious person in Brazil (Padre António Vieira) once said back in the 16th century when criticizing the slavery practice: "If nobody cares about what I preach, I will preach to the fish but will not stay silent". Reminded me of what Richard Stallman once wrote about Ubuntu some time ago and how it still made sense today. I understood better the motivation of Stallman and Vieira to write such words, it is disappointment.
So, feeling overwhelmed with people replying on the forum topic where Unity is great, where the loss of privacy is not true despite recent leaks. I felt quite alone on this question and wrote a message to Richard Stallman. To my surprise, he replied with calming words of advice.
It was a pacifying answer. At that point I just wanted to go back into the discussion, talk loud, do noise and bring to surface that these kind of things are simply not right. His answer is much less conflicting, indeed there is a lot to learned from RMS.When people say, "Nobody cares", you can respond, "Quit exaggerating. Lots of people do care. Neither of us can speak for others. The question is, do YOU care?"
So. Lesson learned. I stopped arguing with those folks on the forum topic after feeling that there was nothing more of productive to be added from my participation. I will nevertheless preach to the fishes with the hope that some day in the future we can have Ubuntu as a "good-guy" again.
Com! magazine article, progress on wb
It has been exactly a month since the new winbuilder generation was released and I already see a screenshot of the software on the most recent edition of the Com! magazine.
Kudos for the editorial team that works so fast! :-)
The next step already in progress is to add support for visually impaired users, following the feedback from users it was possible for me and Peter to make sure that the next editions of winbuilder can be used by users who cannot see.
The choice of using Java has really helped out to make this possible with minimal effort on our side, there was already available a bridge for Windows workstations that allows to tie up directly with the accessibility features. To test, I have installed NVDA on my own computer and simply closed my eyes to get into the skin of those who cannot rely on vision to use a computer. It is not easy, users that struggle with this problem every day have my utmost respect and admiration.
The NVDA software reads out loud what is happening on the screen to the end-user. Since wb is distributing its own version of Java, it was possible for us to slipstream the files required to make the bridge connection work. This way we make winbuilder easier to be interpreted by NVDA.
Things have been so busy recently that I haven't had as much time as desired to do all the things that are needed. Over the past month I've changed the default theme of official website to a MSDOS theme: http://winbuilder.net and then updated some of the content. There is still the need for a big overhaul and to write proper documentation. It is kind of convenient to publish no documentation for the moment since it helped to refrain the motivation of developers to write plugins for a not-yet stable platform, allowing us to make the needed changes/corrections with minimal impact to both developers and end-users.
Packed inside winbuilder is also the phenomenal support for creating VHD archives out-of-the-box, but I've got really no time to go ahead and write projects that demonstrate this feature to create VHD based boot disks. Anyone available to volunteer for the task?
On a side note, UBCD4win has no longer a forum. This was kind of sad to see. Anyone knows what happened?
Lots of work, let's move my friends! :-)
Kudos for the editorial team that works so fast! :-)
The next step already in progress is to add support for visually impaired users, following the feedback from users it was possible for me and Peter to make sure that the next editions of winbuilder can be used by users who cannot see.
The choice of using Java has really helped out to make this possible with minimal effort on our side, there was already available a bridge for Windows workstations that allows to tie up directly with the accessibility features. To test, I have installed NVDA on my own computer and simply closed my eyes to get into the skin of those who cannot rely on vision to use a computer. It is not easy, users that struggle with this problem every day have my utmost respect and admiration.
The NVDA software reads out loud what is happening on the screen to the end-user. Since wb is distributing its own version of Java, it was possible for us to slipstream the files required to make the bridge connection work. This way we make winbuilder easier to be interpreted by NVDA.
Things have been so busy recently that I haven't had as much time as desired to do all the things that are needed. Over the past month I've changed the default theme of official website to a MSDOS theme: http://winbuilder.net and then updated some of the content. There is still the need for a big overhaul and to write proper documentation. It is kind of convenient to publish no documentation for the moment since it helped to refrain the motivation of developers to write plugins for a not-yet stable platform, allowing us to make the needed changes/corrections with minimal impact to both developers and end-users.
Packed inside winbuilder is also the phenomenal support for creating VHD archives out-of-the-box, but I've got really no time to go ahead and write projects that demonstrate this feature to create VHD based boot disks. Anyone available to volunteer for the task?
On a side note, UBCD4win has no longer a forum. This was kind of sad to see. Anyone knows what happened?
Lots of work, let's move my friends! :-)
The new Winbuilder generation
Today was a great day. The new winbuilder generation was made available for the first time to the public.
It is a moment that I'm proud in watching. Was a long walk over over the last 4 years to reach the current state where we are able to provide the first WinPE builder in the world that is fully independent from Windows API.
A lot of work to implement native support for reading/writing:
- WIM archives
- ISO 9660 archives
- Link files (used for shortcuts)
- VHD containers formatted with NTFS
- Registry hives
- the details of Windows .exe files
For the first time, a builder combines all necessary components necessary to create a Windows PE boot disk in a fully independent manner.
Why does this matter?
Because controlling the aspects of building a boot disk introduces an amazing level of quality assurance in regards to the end result. We are now able to predict exactly how the builder will work regardless of the platform underneath.
But the new builder doesn't just introduce platform independence, it brings great features such as user interface abstraction. For the time, we have built an application that simultaneously supports three distinct types of user interfaces straight from the box. You can use the command line, use a normal GUI or even use a web browser if you wish. They are all equally relevant and empowered for controlling winbuilder either from an unattended process, or remotely across another computer somewhere on the network, or from your own desktop as traditional if so you wish.
There would be a lot to talk about in regards to changes, I'd just like to resume some of the other relevant points for the sake of succintness:
I'm really happy. Boot disks are generated in around 3 minutes with no fuss.
If you would like to try it out, it is available at http://reboot.pro/files/file/342-winbuilder/
Have fun!
:)
It is a moment that I'm proud in watching. Was a long walk over over the last 4 years to reach the current state where we are able to provide the first WinPE builder in the world that is fully independent from Windows API.
A lot of work to implement native support for reading/writing:
- WIM archives
- ISO 9660 archives
- Link files (used for shortcuts)
- VHD containers formatted with NTFS
- Registry hives
- the details of Windows .exe files
For the first time, a builder combines all necessary components necessary to create a Windows PE boot disk in a fully independent manner.
Why does this matter?
Because controlling the aspects of building a boot disk introduces an amazing level of quality assurance in regards to the end result. We are now able to predict exactly how the builder will work regardless of the platform underneath.
But the new builder doesn't just introduce platform independence, it brings great features such as user interface abstraction. For the time, we have built an application that simultaneously supports three distinct types of user interfaces straight from the box. You can use the command line, use a normal GUI or even use a web browser if you wish. They are all equally relevant and empowered for controlling winbuilder either from an unattended process, or remotely across another computer somewhere on the network, or from your own desktop as traditional if so you wish.
There would be a lot to talk about in regards to changes, I'd just like to resume some of the other relevant points for the sake of succintness:
- uses java-like language for other developers to write their plugins
- we make available an app-store web service for uploading and distributing the plugins
- plugins use a "hook" system that allows them being triggered according to specific system messages
- settings for plugins use a nice a customizable HTML format
- Windows, Mac OSX and Linux are supported as platforms
- can automatically build a WinPE boot disk without needing user interaction
- does not requires admin permissions
- does not require installing any drivers
- supports full translation of log messages to other languages
I'm really happy. Boot disks are generated in around 3 minutes with no fuss.
If you would like to try it out, it is available at http://reboot.pro/files/file/342-winbuilder/
Have fun!
:)
A trapped Nokia
And so the riddle ends. Microsoft made a deal to buy the Nokia phone division for an incredibly low price of 4 Billion euros.
Might help to note that the current CEO of Nokia was a Microsoft executive just three years ago. When he first arrived, I just hated to see his decision of throwing away the Meego operating system in preference of moving to a Windows phone OS.
You might not remember but Nokia was an early supporter of Linux based operating systems for cellphones, their machines were not only in front of the market as the software itself was already ahead of times.
Microsoft on the other hand, pushed their muscle and made nothing other than Windows CE. An operating system that resembled more of Windows 3.11 than a modern OS for handheld devices. It is sad. Sad that Microsoft never really understood that people have an opinion and a say about their technology preferences. As a big company, for sure they can make life harder on end-users to ensure they get squeezed but you won't get loyalty out of them that way.
It isn't surprising that when a valid alternative surfaces like Android, that people just jump in flocks. Now, through planting a trojan horse as CEO on Nokia and bringing it down to the knees we see MS acquiring the assets of the once largest cell phone manufacturer.
What Microsoft management seems to neglect is that good karma and reputation are based on what you do, these are not things that can easily be bought.
Through this awful chain of events, the former Nokia CEO, Stephen Elop has acquired a awful amount of bad karma. First for destroying the independence of Nokia as a software and hardware manufacturer, second for selling the phone division to Microsoft and jumping happily to their wagon.
Now tell me. Do you really think that people will support a windows phone built with such people in charge? I'd say plenty of analysts will say that people are like sheep which either don't know or don't care about these kind of things. But let me tell you, Nokia as a cellphone maker had a story of their own. Had emotions, had a spirit and a touch that made users feel quite connected to their adventures.
Microsoft has no such thing. Has Ballmer as (ex)CEO that bullies just about everyone and now a pretender to his throne (Elop) that literally buried one of the most shining cellphone makers in the world.
Maybe people are dumb indeed. With Internet access still being relatively free in the current day and age, there is plenty of information and the sympathies will not be high for Microsoft.
The price given for Nokia is a shame indeed. Just this week Verizon bought over half the stocks on their own company that were owned by Vodafone in a deal that was worth over 100Billion. Skype, the chat program bought over by Microsoft was done for a cost of 6 billion.
Not good, not fair for Nokia.
At least Elop is far away from the Finnish company now.
Nokia, how about starting a new phone division?
Might help to note that the current CEO of Nokia was a Microsoft executive just three years ago. When he first arrived, I just hated to see his decision of throwing away the Meego operating system in preference of moving to a Windows phone OS.
You might not remember but Nokia was an early supporter of Linux based operating systems for cellphones, their machines were not only in front of the market as the software itself was already ahead of times.
Microsoft on the other hand, pushed their muscle and made nothing other than Windows CE. An operating system that resembled more of Windows 3.11 than a modern OS for handheld devices. It is sad. Sad that Microsoft never really understood that people have an opinion and a say about their technology preferences. As a big company, for sure they can make life harder on end-users to ensure they get squeezed but you won't get loyalty out of them that way.
It isn't surprising that when a valid alternative surfaces like Android, that people just jump in flocks. Now, through planting a trojan horse as CEO on Nokia and bringing it down to the knees we see MS acquiring the assets of the once largest cell phone manufacturer.
What Microsoft management seems to neglect is that good karma and reputation are based on what you do, these are not things that can easily be bought.
Through this awful chain of events, the former Nokia CEO, Stephen Elop has acquired a awful amount of bad karma. First for destroying the independence of Nokia as a software and hardware manufacturer, second for selling the phone division to Microsoft and jumping happily to their wagon.
Now tell me. Do you really think that people will support a windows phone built with such people in charge? I'd say plenty of analysts will say that people are like sheep which either don't know or don't care about these kind of things. But let me tell you, Nokia as a cellphone maker had a story of their own. Had emotions, had a spirit and a touch that made users feel quite connected to their adventures.
Microsoft has no such thing. Has Ballmer as (ex)CEO that bullies just about everyone and now a pretender to his throne (Elop) that literally buried one of the most shining cellphone makers in the world.
Maybe people are dumb indeed. With Internet access still being relatively free in the current day and age, there is plenty of information and the sympathies will not be high for Microsoft.
The price given for Nokia is a shame indeed. Just this week Verizon bought over half the stocks on their own company that were owned by Vodafone in a deal that was worth over 100Billion. Skype, the chat program bought over by Microsoft was done for a cost of 6 billion.
Not good, not fair for Nokia.
At least Elop is far away from the Finnish company now.
Nokia, how about starting a new phone division?
An MSDOS theme for Wordpress
I don't know where to use this theme right now but I'm writing a blog post so that more folks can find it: http://wordpress.org/themes/wp386
A great looking MSDOS theme. Call it "DOStalgy" but I sometimes really miss those days.
A great looking MSDOS theme. Call it "DOStalgy" but I sometimes really miss those days.
The rebirth of SourceForge
Sourceforge has been quite a presence in my life, it is the place where eventually I ended up visiting way too often across the years whenever in need of downloading some file from an open source project.
One thing that always bugged me was the fact that the site itself was quirk, slow and just plain difficult to use for both developers and end-users.
Now, I'm getting pleasantly surprised (and perhaps spoiled). Slowly, very slowly I see a new Sourceforge surfacing. It accepts logins from the most popular web services available today (google, facebook, twitter, ...), has a front page that actually looks useful and inviting for visitors to use. Last but not least, finally seems to be focused in improving the forum support. Check it out at https://sourceforge.net/
Bravo! I have to say that this is a rebirth for this giant.
One thing that always bugged me was the fact that the site itself was quirk, slow and just plain difficult to use for both developers and end-users.
Now, I'm getting pleasantly surprised (and perhaps spoiled). Slowly, very slowly I see a new Sourceforge surfacing. It accepts logins from the most popular web services available today (google, facebook, twitter, ...), has a front page that actually looks useful and inviting for visitors to use. Last but not least, finally seems to be focused in improving the forum support. Check it out at https://sourceforge.net/
Bravo! I have to say that this is a rebirth for this giant.
Virtual obsolescence
This is a topic that worries me. I guess few other people will have interest in planning ahead what happens to software creations/property/art when they become obsolete and depend on some obsolete/discontinued component to work but this is an eventual fact of software and hardware life.
So, why should you worry?
Well, for a start you will be throwing down the trash all the money, effort and resources that you already acquired up to that point and then repeat it all over again.
Say for example that we are in 1990 and you just bought a new IBM personal computer or acquired yourself a nice website domain. After a couple of years you see no more use for any of them and they quickly get disposed with something more interesting at that moment.
What is the result? The computer or website domain that you acquired might have cost you some hundreds to thousands of dollars and now it is just plain trash in your eyes, quickly lost from sight and only a memory to you.
Now what happens when fast forwarding 30 years from now? That old piece of hardware might possibly hold now a status of vintage and the website domain gets its value increased due to longevity of its registration date which is a factor of value to collectors.
I'm not advocating that you should keep everything obsolete around your house, the point is asking you to think and apply this concept to the software bits that you write, the work that results from your own intellectual creations which many times will be lost after a mere years.
In this virtual world, you can likely adopt two distinct approaches: 1) accept that things might be lost forever and do nothing or 2) accept that things might be lost but do something to counter-act this kind of obsolescence.
If you're the type of person willing to follow option 2) then here is my list of recommendations to consider.
That's it, three tips to reduce the obsolescence risk and safeguard a piece of the past, today.
This kind of thinking-ahead requires additional work, expenses and effort. In the end, only pays off when someone else finds your effort useful. Even then it is certainly unpredictable what might happen. Still, who knows if you'll do something today that will help someone else 2000 years from now like the Rosetta Stone did. Unfortunately for us geeks it gets difficult to write bytes in stones, creativity will certainly be needed to solve this challenge.. :-)
What about you? What kind of virtual footprint will you leave for the future?
So, why should you worry?
Well, for a start you will be throwing down the trash all the money, effort and resources that you already acquired up to that point and then repeat it all over again.
Say for example that we are in 1990 and you just bought a new IBM personal computer or acquired yourself a nice website domain. After a couple of years you see no more use for any of them and they quickly get disposed with something more interesting at that moment.
What is the result? The computer or website domain that you acquired might have cost you some hundreds to thousands of dollars and now it is just plain trash in your eyes, quickly lost from sight and only a memory to you.
Now what happens when fast forwarding 30 years from now? That old piece of hardware might possibly hold now a status of vintage and the website domain gets its value increased due to longevity of its registration date which is a factor of value to collectors.
I'm not advocating that you should keep everything obsolete around your house, the point is asking you to think and apply this concept to the software bits that you write, the work that results from your own intellectual creations which many times will be lost after a mere years.
In this virtual world, you can likely adopt two distinct approaches: 1) accept that things might be lost forever and do nothing or 2) accept that things might be lost but do something to counter-act this kind of obsolescence.
If you're the type of person willing to follow option 2) then here is my list of recommendations to consider.
- Style doesn't go out of fashion. Pick a domain name that you are proud about right now and keep it for as long as you can. In some years the domain might be unused but if you have chosen a name you personally fell attached, then it might still be used for other projects or just kept as a collector item (with added value if it hosted a site in the past). As for hardware, try to pick a good looking machine. Extra points if it is sturdy and from a reputable vendor. The odds are that you will remain a proud owner of the machine regardless of how obsolete the hardware underneath has became in the meanwhile (for e.g. I kept religiously my first laptop from the 90's and 20 years later was a portion of computer history and my own history).
- Use public hosting whenever possible. Say for example this blog, Google will keep my words around for thousands of moon-cycles after I stop walking on this earth. Invest your time wisely in choosing a network platform that has conditions to last. A private hosting eventually gets disconnected or some public services will not care about you and delete everything after a few years (for e.g. Microsoft already deleted my first blog, GitHub will delete your account if unused, Yahoo killed Geocities, ...). At least with companies like Google I will be sure that these blog memories will live forward since they rely on data gathering as a business model.
- Share your projects. If you're founding/maintaining a project that you hold dear, then consider opening your hand of the sole ownership. Let other people of your trust to step aboard as administrators and owners instead of just grouping followers or supporters. Being a creative genie is a fabulous thing but when you're doing everything alone then alone you remain. For me (as author and/or user), it hurts when one of these projects fades to oblivion after left alone. This model of work might function good for someone like Fabrice Bellard, but gosh, just share your projects. It is a great thing for everyone if you can walk away one day and see your child becoming fabulous as it grows through the years.
That's it, three tips to reduce the obsolescence risk and safeguard a piece of the past, today.
This kind of thinking-ahead requires additional work, expenses and effort. In the end, only pays off when someone else finds your effort useful. Even then it is certainly unpredictable what might happen. Still, who knows if you'll do something today that will help someone else 2000 years from now like the Rosetta Stone did. Unfortunately for us geeks it gets difficult to write bytes in stones, creativity will certainly be needed to solve this challenge.. :-)
What about you? What kind of virtual footprint will you leave for the future?
Hello 2013!
Here we are at the verge of brand new year.
For me, Christmas 2011 still feels like yesterday and 2012 was deleted swiftly like a big ISO file sitting on a fast USB flash drive. It is odd but I'm actually not looking so forward in going through 2013. Last year was crazy in terms of events, the year before that one was no different and for a change would be nice to get some peace and quiet for a while.
Looking back I can say that In overall things could be worse, much worse. But as someone else rightfully told me today: aiming to reach "not worse" is quite simply "not good". We have to aim high and do better.
So, I'm not aiming for 2013. Time passes too fast for plans or meaningful resolutions so I am quickly saying hello to this year and already looking forward to what we can better on the next one.
Apart from the philosophical matters, be happy. Enjoy a great year with your family, friends and projects. Be happy and I wish you a feliz 2013!
For me, Christmas 2011 still feels like yesterday and 2012 was deleted swiftly like a big ISO file sitting on a fast USB flash drive. It is odd but I'm actually not looking so forward in going through 2013. Last year was crazy in terms of events, the year before that one was no different and for a change would be nice to get some peace and quiet for a while.
Looking back I can say that In overall things could be worse, much worse. But as someone else rightfully told me today: aiming to reach "not worse" is quite simply "not good". We have to aim high and do better.
So, I'm not aiming for 2013. Time passes too fast for plans or meaningful resolutions so I am quickly saying hello to this year and already looking forward to what we can better on the next one.
Apart from the philosophical matters, be happy. Enjoy a great year with your family, friends and projects. Be happy and I wish you a feliz 2013!
Stubborn Ubuntu
There we go. On the eve of an announcement at Ubuntu without any reaction on the general hate from the Ubuntu community to the "unity" desktop environment that causes so much division.
Can we for a change admit that Unity is wrong? It's crap. If the best that an average user can say about this environment is that they "got used" to it, then this is just plain wrong and you're being too stubborn for doing it right.
A good environment either gives you the "wow" effect or lets you get things done. Unity does neither. Two years have passed and this crappy alternative to the gnome environment is still in practice. No significant improvements, just crap. Browse by yourself the Ubuntu forum, the personal blog of Mark and check out the reactions. I'm really not alone on this frustration, just wish we could still have a usable OS.
An announcement will soon be made and I just wish it was announcing that the desktop is returning to the time when we could use it.
Seriously, run a pool of opinion to the community. Ask us for our educated opinion and let the numbers drive the market. Going for a fancy touch-based UI is not a thing that has to be forced onto you as no other remedy, this should be something that people want to embrace today. Ubuntu is doing it wrong, please change.
Can we for a change admit that Unity is wrong? It's crap. If the best that an average user can say about this environment is that they "got used" to it, then this is just plain wrong and you're being too stubborn for doing it right.
A good environment either gives you the "wow" effect or lets you get things done. Unity does neither. Two years have passed and this crappy alternative to the gnome environment is still in practice. No significant improvements, just crap. Browse by yourself the Ubuntu forum, the personal blog of Mark and check out the reactions. I'm really not alone on this frustration, just wish we could still have a usable OS.
An announcement will soon be made and I just wish it was announcing that the desktop is returning to the time when we could use it.
Seriously, run a pool of opinion to the community. Ask us for our educated opinion and let the numbers drive the market. Going for a fancy touch-based UI is not a thing that has to be forced onto you as no other remedy, this should be something that people want to embrace today. Ubuntu is doing it wrong, please change.
Subscribe to:
Posts (Atom)