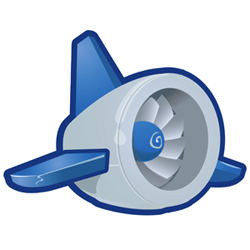
Yesterday I've created my first application using Google App Engine.
I've been thinking about the possibilities of using a cloud computing environment for quite some time now. Google brings the advantage that you can use their services for free up to a given threshold of resources.
When I first heard about google app engine, it was some years ago and it was only supported on Python. At the time, phyton didn't exactly rock my world so I just walked away and kept on doing other things.
A few months ago, Java support was announced. My wild guess is that Phyton was not exactly a popular choice amongst many developers but Java is a whole different case.
So, I went on with my trial of their (free) service.
The Java environment that is officially supported by Google is based on the Eclipse IDE, since my preferences on coding fall more on the NetBeans side of life, I've "googled" for this alternative.
It turns out to be a quite simple process of configuration.
The first step is downloading and unpacking the Google App Engine SDK to some folder inside your disk - http://code.google.com/intl/en/appengine/downloads.html
No fancy install involved, just unzip and place it somewhere nice.
-------------------
Then, we open NetBeans. Google support is added as a plugin for the NetBeans IDE. There is a very good page that details these steps: http://kenai.com/projects/nbappengine/pages/NBInstall
When configuring the google plugin, I didn't selected the Hibernate Support and didn't seemed to matter much in either case.
After all said and done, you're only missing to add google app engine as a server inside your environment and this is also a simple task.
Click on the "Services" tab (that is next to "Projects" and "Files" on the right side of the IDE) and right click on the "Servers" item.
All that is missing is add a new server - select the "Google App Engine".
---------------
You should now have everything set up.
To start a web project, create a new project and select "Java Web" --> "Web Application". It will create you a template project that will be saying "Hello world!"
When you're ready to publish the project on the cloud computing environment, just right click on the project and choose "Deploy to google app engine".
Don't forget that you need to have a project already configured on the side of the google app website. After this you should be able to visit your URL and try out the results.
You can also view a full video of all these steps at the following location: http://kenai.com/projects/nbappengine/pages/Home
---------
Hope you have fun with cloud computing, this is a really simple and interesting concept at a very affordable cost.
:)